從零開始使用Golang構(gòu)建高質(zhì)量的命令行應(yīng)用程序
從零開始使用Golang構(gòu)建高質(zhì)量的命令行應(yīng)用程序
命令行應(yīng)用程序是一個非常有用的工具,可以在終端中執(zhí)行各種操作,如查看文件,運行腳本等等。在本文中,我們將介紹如何使用Golang構(gòu)建高質(zhì)量的命令行應(yīng)用程序。
技術(shù)準備
在開始之前,我們需要安裝Golang并設(shè)置好環(huán)境變量。在命令行中輸入以下命令來檢查是否安裝成功:
go version
如果顯示了Golang的版本號,那么說明已經(jīng)安裝成功。
接下來,我們將使用以下兩個Golang庫來幫助構(gòu)建命令行應(yīng)用程序:
1. Cobra:Cobra是一個用于創(chuàng)建命令行應(yīng)用程序的庫。它提供了創(chuàng)建命令、子命令、標記和其他功能的API。
2. Viper:Viper是一個用于處理配置文件和命令行標記的庫。它提供了多種格式的配置文件支持,例如JSON、YAML、TOML等。
安裝這兩個庫非常簡單。在命令行中,使用以下命令安裝Cobra和Viper:
go get -u github.com/spf13/cobra/cobrago get -u github.com/spf13/viper/viper
接下來,我們將使用這兩個庫來構(gòu)建一個簡單的命令行應(yīng)用程序。
創(chuàng)建項目
在開始之前,我們需要創(chuàng)建一個新的Golang項目。在命令行中,輸入以下命令來創(chuàng)建一個名為“mycli”的新項目:
mkdir myclicd mycligo mod init mycli
在創(chuàng)建了項目之后,我們需要創(chuàng)建一個新的命令行應(yīng)用程序。
使用以下命令創(chuàng)建一個名為“mycmd”的新命令:
cobra init --pkg-name mycmd
這將創(chuàng)建一個名為“mycmd”的新目錄,并在其中創(chuàng)建一個名為“cmd”的新目錄。
mycli/ ├── cmd/ │ └── mycmd/ │ ├── root.go │ └── mycmd.go ├── go.mod └── main.go
在“mycmd”目錄中,我們可以看到兩個文件:root.go和mycmd.go。
root.go文件是應(yīng)用程序的入口文件。它包含了一些初始化代碼,例如創(chuàng)建命令和添加標記。
mycmd.go文件是我們要創(chuàng)建的命令文件。它將包含我們的業(yè)務(wù)邏輯和處理邏輯。
創(chuàng)建命令
在開始編寫代碼之前,我們需要先創(chuàng)建一個名為“hello”的新命令。
使用以下命令在mycmd.go中創(chuàng)建一個名為“hello”的新命令:
cobra add hello
這將在mycmd目錄中創(chuàng)建一個名為“hello.go”的新文件,并將新命令添加到root.go中。
mycli/ ├── cmd/ │ └── mycmd/ │ ├── root.go │ ├── hello.go // 新增的文件 │ └── mycmd.go ├── go.mod └── main.go
在hello.go文件中,我們可以看到一個名為“helloCmd”的新結(jié)構(gòu)體。這個結(jié)構(gòu)體代表了我們的新命令。
我們可以使用以下代碼為命令添加一些元數(shù)據(jù):
func init() { helloCmd.PersistentFlags().String("name", "world", "A name to say hello to.") rootCmd.AddCommand(helloCmd)}
這里我們添加了一個名為“name”的標記,并設(shè)置了默認值為“world”。
接下來,我們需要編寫一些代碼來處理我們的命令。
處理命令
在hello.go文件中,我們可以看到一個名為“runHello”的新函數(shù)。這個函數(shù)是我們的業(yè)務(wù)邏輯。
下面是代碼實現(xiàn):
func runHello(cmd *cobra.Command, args string) { name, _ := cmd.Flags().GetString("name") fmt.Printf("Hello, %s!\n", name)}
這個函數(shù)從標記中獲取名稱,并使用fmt包來打印“Hello,name!”的消息。
在main.go文件中,我們可以看到一個名為“Execute”的函數(shù)。這個函數(shù)是應(yīng)用程序的入口點。
我們只需要在這個函數(shù)中添加以下代碼即可:
if err := mycmd.Execute(); err != nil { fmt.Println(err) os.Exit(1)}
這個代碼將執(zhí)行應(yīng)用程序,并處理任何錯誤。現(xiàn)在,我們已經(jīng)完成了一個簡單的命令行應(yīng)用程序。
完整代碼如下所示:
mycmd/cmd/hello.go:
package cmd
import (
"fmt"
"github.com/spf13/cobra"
)
var helloCmd = &cobra.Command{
Use: "hello",
Short: "Say hello",
Run: runHello,
}
func init() { helloCmd.PersistentFlags().String("name", "world", "A name to say hello to.") rootCmd.AddCommand(helloCmd)}
func runHello(cmd *cobra.Command, args string) { name, _ := cmd.Flags().GetString("name") fmt.Printf("Hello, %s!\n", name)}
mycmd/cmd/root.go:
package cmdimport ( "fmt" "os" "github.com/spf13/cobra")var rootCmd = &cobra.Command{ Use: "mycmd", Short: "A brief description of your application", Long: A longer description that spans multiple lines and likely contains examples and usage of using your application. For example: Cobra is a CLI library for Go that empowers applications. This application is a tool to generate the needed files to quickly create a Cobra application., Run: func(cmd *cobra.Command, args string) { // Do Stuff Here fmt.Println("Welcome to mycmd!") },}func init() { cobra.OnInitialize(initConfig) rootCmd.PersistentFlags().StringVar(&cfgFile, "config", "", "config file (default is $HOME/.mycmd.yaml)") rootCmd.Flags().BoolP("toggle", "t", false, "Help message for toggle")}func initConfig() { if cfgFile != "" { // Use config file from the flag. viper.SetConfigFile(cfgFile) } else { // Find home directory. home, err := homedir.Dir() if err != nil { fmt.Println(err) os.Exit(1) } // Search config in home directory with name ".mycmd" (without extension). viper.AddConfigPath(home) viper.SetConfigName(".mycmd") } viper.AutomaticEnv() // If a config file is found, read it in. if err := viper.ReadInConfig(); err == nil { fmt.Println("Using config file:", viper.ConfigFileUsed()) }}
mycmd/main.go:
package mainimport "mycli/cmd"func main() { if err := cmd.Execute(); err != nil { panic(err) }}
多格式配置文件支持
在上面的代碼中,我們使用了硬編碼的標記來設(shè)置“name”屬性。但是,在實際應(yīng)用程序中,我們通常會使用配置文件來設(shè)置標記。
Viper庫支持多種格式的配置文件,例如JSON、YAML、TOML等。我們可以使用以下代碼來初始化Viper:
func initConfig() { if cfgFile != "" { // Use config file from the flag. viper.SetConfigFile(cfgFile) } else { // Find home directory. home, err := homedir.Dir() if err != nil { fmt.Println(err) os.Exit(1) } // Search config in home directory with name ".mycmd" (without extension). viper.AddConfigPath(home) viper.SetConfigName(".mycmd") } viper.AutomaticEnv() // If a config file is found, read it in. if err := viper.ReadInConfig(); err == nil { fmt.Println("Using config file:", viper.ConfigFileUsed()) }}
這個代碼將首先查找命令行標記中的配置文件,并在找到時使用它。否則,它將查找用戶主目錄中名為“mycmd”的文件。
以下是我們可以使用的兩個示例配置文件:
JSON:
{ "hello": { "name": "Gopher" }}
YAML:
hello: name: Gopher
我們可以使用以下代碼在應(yīng)用程序中讀取這些配置:
name := viper.GetString("hello.name")
結(jié)論
在本文中,我們已經(jīng)學習了如何使用Cobra和Viper構(gòu)建高質(zhì)量的命令行應(yīng)用程序。我們了解了如何創(chuàng)建命令、添加標記、處理命令和處理配置文件。希望這些知識能夠幫助你快速構(gòu)建出自己的命令行應(yīng)用程序。

猜你喜歡LIKE
相關(guān)推薦HOT
更多>>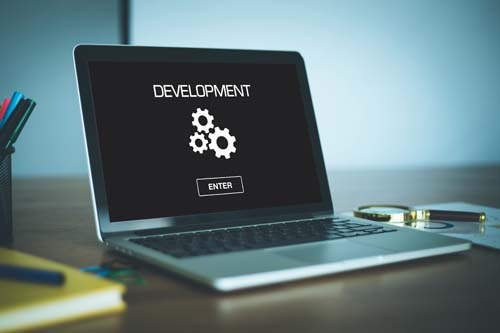
黑客攻擊的常見手段?網(wǎng)絡(luò)安全專家教你如何一步一步防范
黑客攻擊的常見手段?網(wǎng)絡(luò)安全專家教你如何一步一步防范網(wǎng)絡(luò)攻擊惡化日益,黑客們的手段也越來越高超,防范這些攻擊成為了網(wǎng)絡(luò)安全工作者必備的...詳情>>
2023-12-27 19:02:49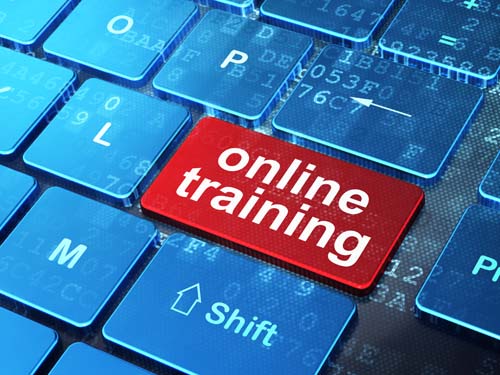
網(wǎng)絡(luò)攻防實驗室的建設(shè)與運營
網(wǎng)絡(luò)攻防實驗室的建設(shè)與運營隨著網(wǎng)絡(luò)攻擊的不斷增多,網(wǎng)絡(luò)安全已經(jīng)變得越來越重要。一個好的網(wǎng)絡(luò)攻防實驗室不僅有助于提高學生的技能和知識,還...詳情>>
2023-12-27 14:14:49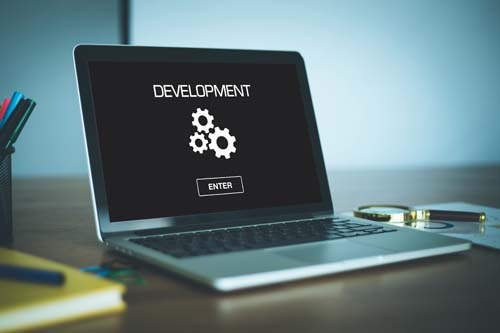
如何構(gòu)建一個安全的密碼策略
如何構(gòu)建一個安全的密碼策略在現(xiàn)今信息時代,安全性是至關(guān)重要的。在很多情況下,密碼是保護我們個人信息和公司敏感數(shù)據(jù)的首要防線。因此,構(gòu)建...詳情>>
2023-12-27 13:02:48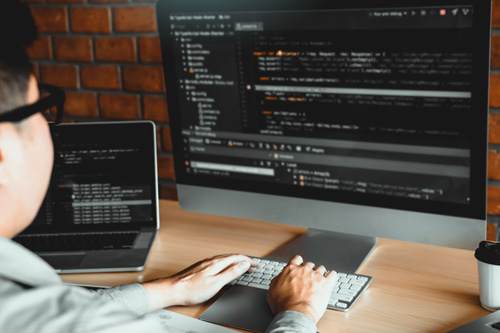
5個有效防范網(wǎng)絡(luò)釣魚的技巧
網(wǎng)絡(luò)釣魚已經(jīng)成為了網(wǎng)絡(luò)安全領(lǐng)域中的嚴重問題,攻擊者通過發(fā)送誘騙性的郵件或鏈接,試圖讓受害者泄露敏感信息。因此,如何有效防范網(wǎng)絡(luò)釣魚攻擊...詳情>>
2023-12-27 11:50:48熱門推薦
如何為網(wǎng)絡(luò)安全做好逆向工程
沸軟件漏洞會帶來哪些安全隱患
熱網(wǎng)絡(luò)安全威脅分析與應(yīng)對指南
熱大數(shù)據(jù)時間下的網(wǎng)絡(luò)安全挑戰(zhàn):如何應(yīng)對日益增長的風險?
新黑客攻擊的常見手段?網(wǎng)絡(luò)安全專家教你如何一步一步防范
暴力破解密碼真的那么可怕嗎?看看這些加密算法就知道了
如何保持網(wǎng)絡(luò)系統(tǒng)的安全性:詳細分析常見的網(wǎng)絡(luò)攻擊方式
深度學習在網(wǎng)絡(luò)安全中的應(yīng)用
網(wǎng)絡(luò)攻防實驗室的建設(shè)與運營
如何構(gòu)建一個安全的密碼策略
5個有效防范網(wǎng)絡(luò)釣魚的技巧
如何截獲和解密SSL流量?
網(wǎng)絡(luò)安全事件響應(yīng)與處置流程
5個提高企業(yè)網(wǎng)絡(luò)安全的技巧
技術(shù)干貨
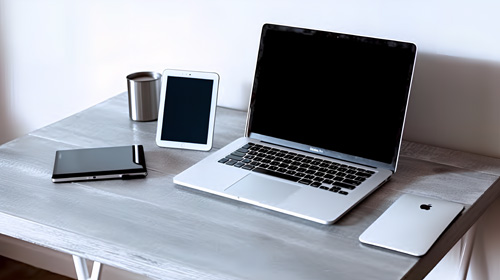
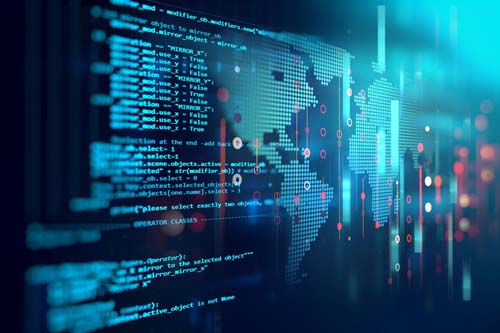
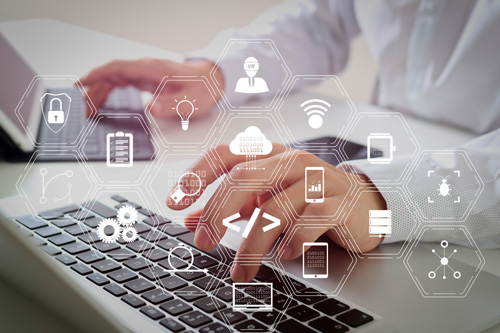
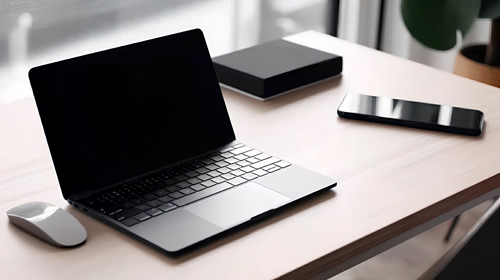
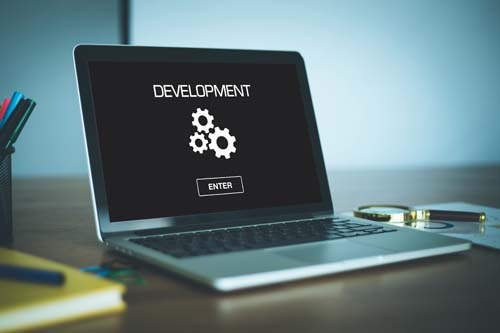
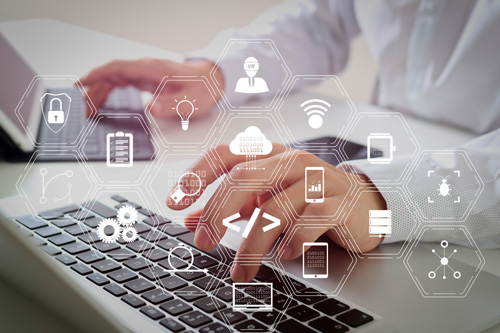
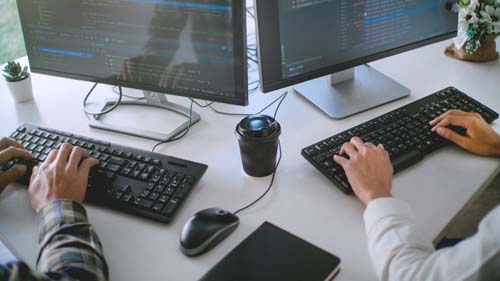
快速通道 更多>>
-
課程介紹
點擊獲取大綱 -
就業(yè)前景
查看就業(yè)薪資 -
學習費用
了解課程價格 -
優(yōu)惠活動
領(lǐng)取優(yōu)惠券 -
學習資源
領(lǐng)3000G教程 -
師資團隊
了解師資團隊 -
實戰(zhàn)項目
獲取項目源碼 -
開班地區(qū)
查看來校路線